代码
#region 获取C#属性对应的MySql数据类型
/// <summary>
/// 获取C#属性对应的MySql数据类型
/// </summary>
/// <param name="propertyInfo">属性</param>
/// <returns></returns>
private static string GetMySqlTypeStrWithPropertyInfo(PropertyInfo propertyInfo)
{
string result = string.Empty;
string typeName = propertyInfo.PropertyType.FullName ?? "";
bool canNull = false;
if (propertyInfo.PropertyType.Name.Contains(nameof(Nullable)))
{
var t = propertyInfo.PropertyType.GenericTypeArguments.FirstOrDefault();
if (t != null)
{
typeName = t.FullName ?? "";
canNull = true;
}
}
var attr = propertyInfo.GetCustomAttribute<StringLengthAttribute>();
if (attr != null)
{
int maxLength = attr.MaximumLength;
result = maxLength <= 0 ? "Text" : $"VARCHAR({maxLength})";
}
else
{
//枚举处理
if (propertyInfo.PropertyType.BaseType != null && !string.IsNullOrWhiteSpace(propertyInfo.PropertyType.BaseType.FullName) && propertyInfo.PropertyType.BaseType.FullName.Contains("System.Enum"))
{
var fields = propertyInfo.PropertyType.GetFields(BindingFlags.Static | BindingFlags.Public);
List<string> enumList = new List<string>();
foreach (var fi in fields)
enumList.Add(fi.Name);
typeName = $"enum('{string.Join("','", enumList)}')";
}
typeName = typeName.Replace("System.", "");
result = GetMySqlTypeStr(typeName);
}
result += $"{(canNull ? " NULL" : " NOT NULL")}";
return result;
}
#endregion
#region C#数据类型转换为MySQL列数据类型
/// <summary>
/// C#数据类型转换为MySQL列数据类型
/// </summary>
/// <param name="TypeName">属性类型名称</param>
/// <returns></returns>
private static string GetMySqlTypeStr(string TypeName)
{
string result = string.Empty;
switch (TypeName)
{
case nameof(System.Boolean):
result = "BOOL";
break;
case nameof(System.Int32):
result = "INT";
break;
case nameof(System.Single):
result = "FLOAT";
break;
case nameof(System.Double):
result = "DOUBLE";
break;
case nameof(System.Decimal):
result = "DECIMAL";
break;
case nameof(System.String):
result = "VARCHAR(255)";
break;
case nameof(System.DateTime):
result = "DateTime";
break;
case nameof(System.Guid):
result = "CHAR(36)";
break;
default:
result = TypeName;
break;
}
return result;
}
#endregion
测试结果
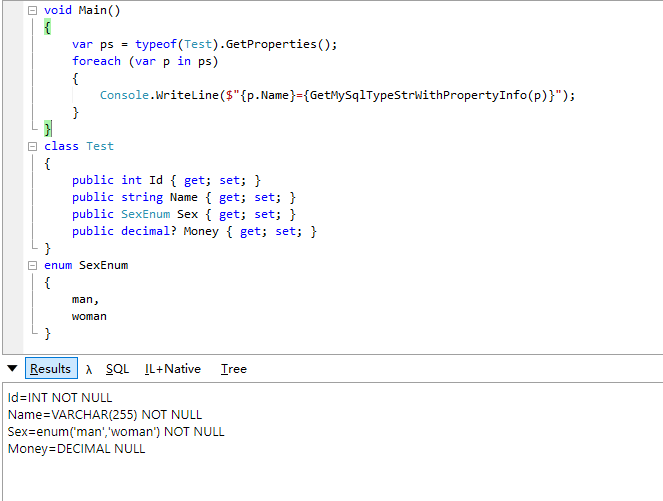
评论区